188 lines
5.0 KiB
Markdown
188 lines
5.0 KiB
Markdown
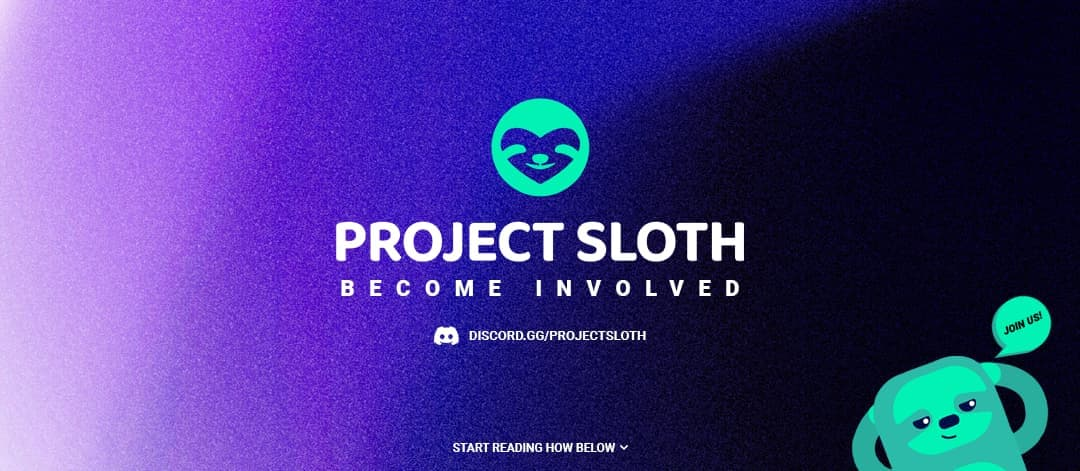
|
|
### Project Sloth UI
|
|
A single resource made up of several smaller, UI-related scripts for use in your server, accessible via client-side exports. Documentation and previews of each are provided below. We will continue to add to this collection in the future.
|
|
|
|

|
|
|
|
## Circle Minigame
|
|
|
|
```
|
|
exports['ps-ui']:Circle(function(success)
|
|
if success then
|
|
print("success")
|
|
else
|
|
print("fail")
|
|
end
|
|
end, 2, 20) -- NumberOfCircles, MS
|
|
```
|
|
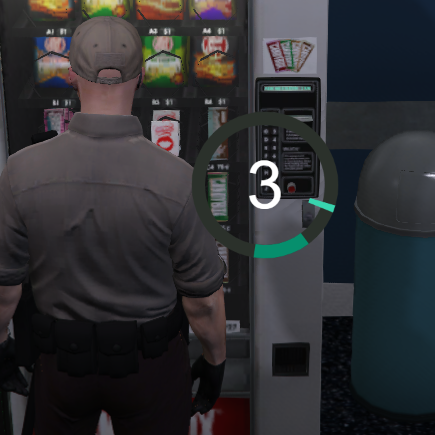
|
|
|
|
## Number Maze
|
|
```
|
|
exports['ps-ui']:Maze(function(success)
|
|
if success then
|
|
print("success")
|
|
else
|
|
print("fail")
|
|
end
|
|
end, 20) -- Hack Time Limit
|
|
```
|
|
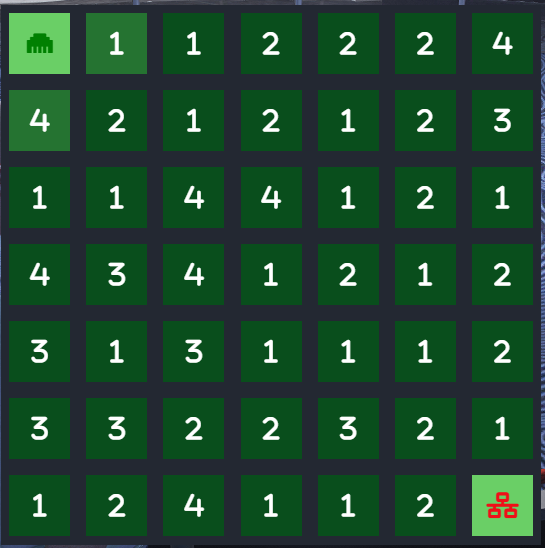
|
|
|
|
## VAR
|
|
```
|
|
exports['ps-ui']:VarHack(function(success)
|
|
if success then
|
|
print("success")
|
|
else
|
|
print("fail")
|
|
end
|
|
end, 2, 3) -- Number of Blocks, Time (seconds)
|
|
```
|
|
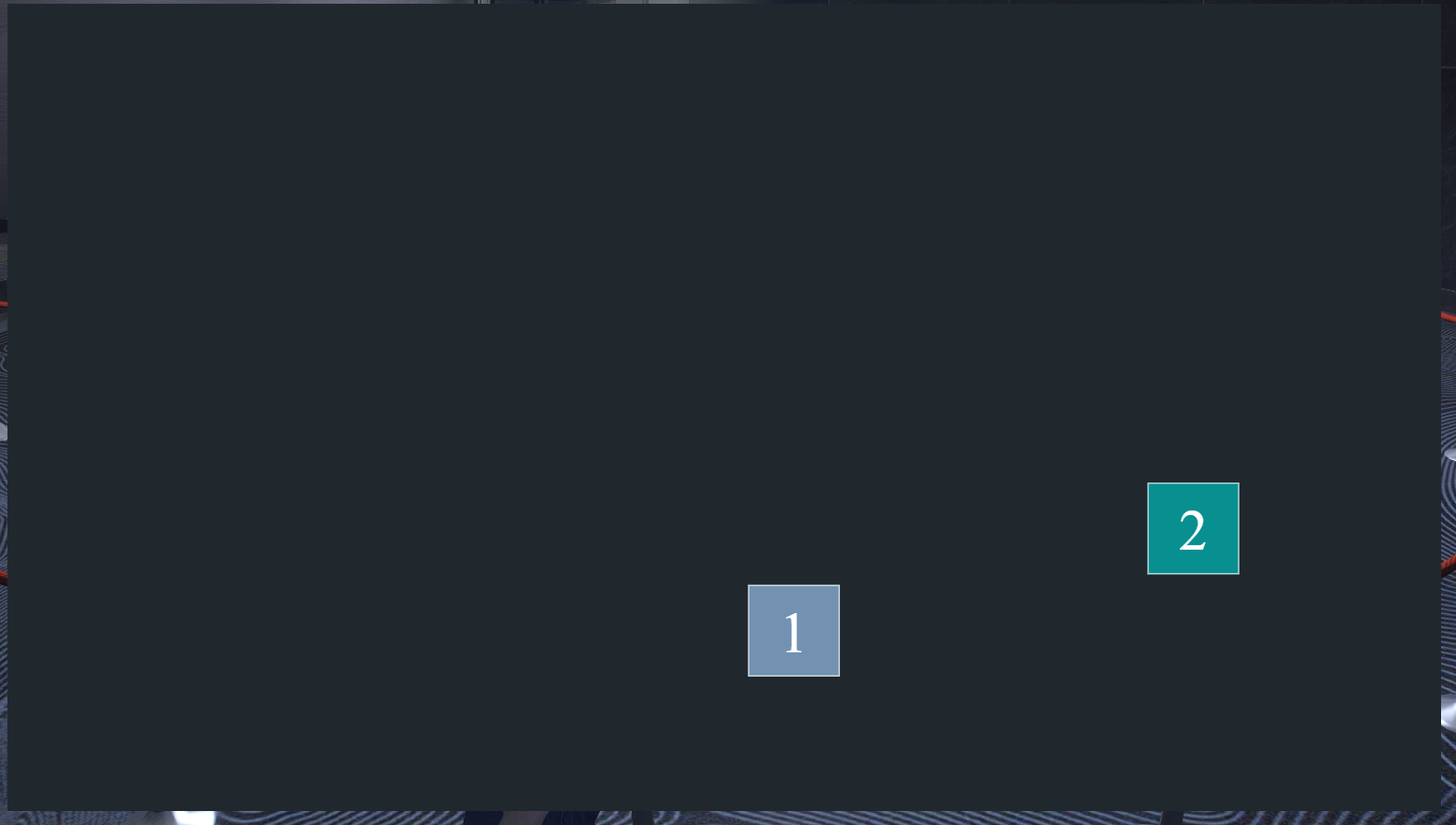
|
|
|
|
## Thermite
|
|
```
|
|
exports['ps-ui']:Thermite(function(success)
|
|
if success then
|
|
print("success")
|
|
else
|
|
print("fail")
|
|
end
|
|
end, 10, 5, 3) -- Time, Gridsize (5, 6, 7, 8, 9, 10), IncorrectBlocks
|
|
```
|
|
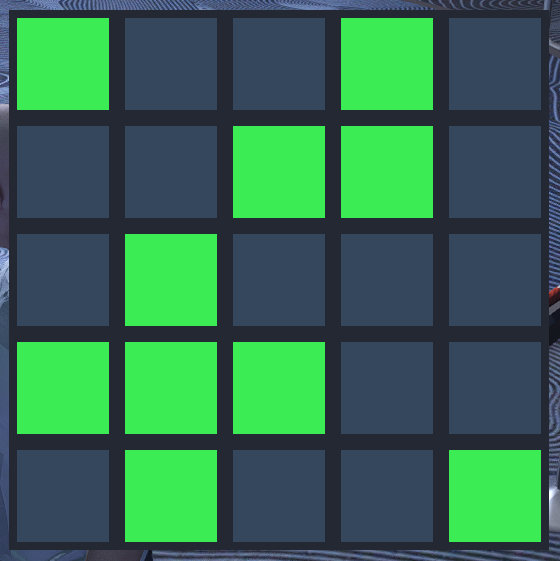
|
|
|
|
## Scrambler
|
|
```
|
|
exports['ps-ui']:Scrambler(function(success)
|
|
if success then
|
|
print("success")
|
|
else
|
|
print("fail")
|
|
end
|
|
end, "numeric", 30, 0) -- Type (alphabet, numeric, alphanumeric, greek, braille, runes), Time (Seconds), Mirrored (0: Normal, 1: Normal + Mirrored 2: Mirrored only )
|
|
```
|
|
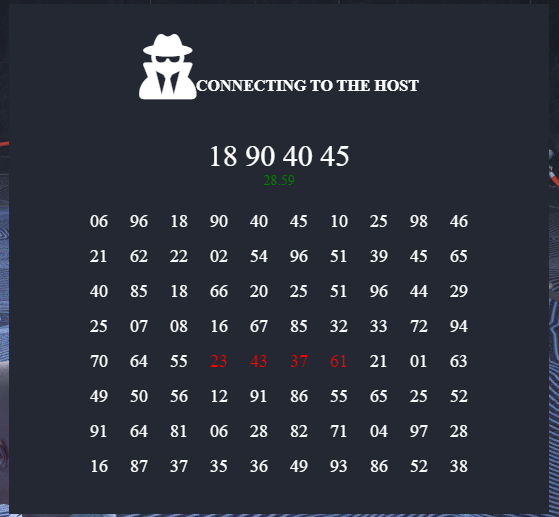
|
|
|
|
## Display Text
|
|
```
|
|
exports['ps-ui']:DisplayText("Example Text", "primary") -- Colors: primary, error, success, warning, info, mint
|
|
exports['ps-ui']:HideText()
|
|
```
|
|
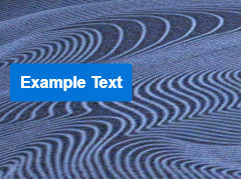
|
|
|
|
## Status UI
|
|
```
|
|
exports['ps-ui']:StatusShow("Area Dominance", {
|
|
"Gang: Ballas",
|
|
"Influence: %100",
|
|
})
|
|
exports['ps-ui']:StatusUpdate("Area Dominance", {
|
|
"Gang: Ballas",
|
|
"Influence: %99",
|
|
})
|
|
exports['ps-ui']:StatusHide()
|
|
```
|
|
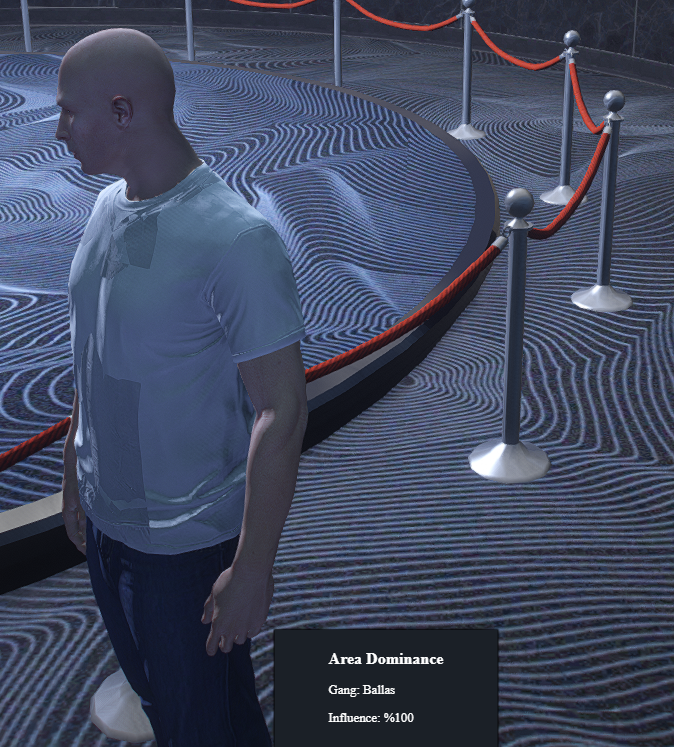
|
|
|
|
## Menus
|
|
```
|
|
exports['ps-ui']:CreateMenu({
|
|
{
|
|
header = "header1",
|
|
text = "text1",
|
|
icon = "fa-solid fa-circle",
|
|
color = "red",
|
|
event = "event:one",
|
|
args = {
|
|
1,
|
|
"two",
|
|
"3",
|
|
},
|
|
server = false,
|
|
|
|
},
|
|
{
|
|
header = "header2",
|
|
text = "text3",
|
|
icon = "fa-solid fa-circle",
|
|
color = "blue",
|
|
event = "event:two",
|
|
args = {
|
|
1,
|
|
"two",
|
|
"3",
|
|
},
|
|
server = false,
|
|
},
|
|
{
|
|
header = "header3",
|
|
text = "text3",
|
|
icon = "fa-solid fa-circle",
|
|
color = "green",
|
|
event = "event:three",
|
|
args = {
|
|
1,
|
|
"two",
|
|
"3",
|
|
},
|
|
server = true,
|
|
},
|
|
{
|
|
header = "header4",
|
|
text = "text4",
|
|
event = "event:four",
|
|
args = {
|
|
1,
|
|
"two",
|
|
"3",
|
|
},
|
|
},
|
|
})
|
|
```
|
|
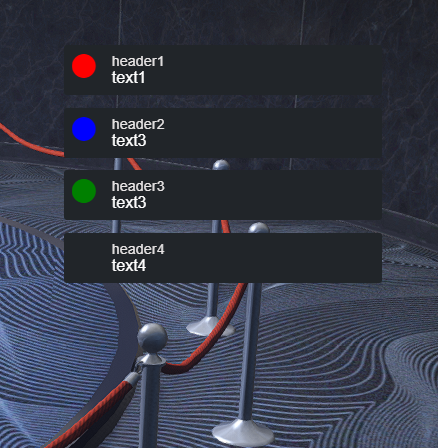
|
|
|
|
## Input
|
|
|
|
```
|
|
local input = exports['ps-ui']:Input({
|
|
title = "Test",
|
|
inputs = {
|
|
{
|
|
type = "text",
|
|
placeholder = "test2"
|
|
},
|
|
{
|
|
type = "password",
|
|
placeholder = "password"
|
|
},
|
|
{
|
|
type = "number",
|
|
placeholder = "666"
|
|
},
|
|
}
|
|
})
|
|
```
|
|
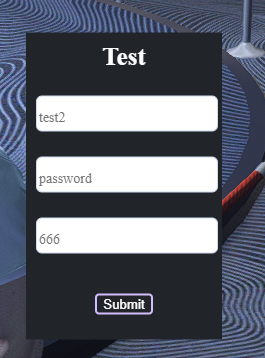
|
|
|
|
## Show Image
|
|
|
|
```
|
|
exports['ps-ui']:ShowImage("https://user-images.githubusercontent.com/91661118/168956591-43462c40-e7c2-41af-8282-b2d9b6716771.png")
|
|
```
|
|
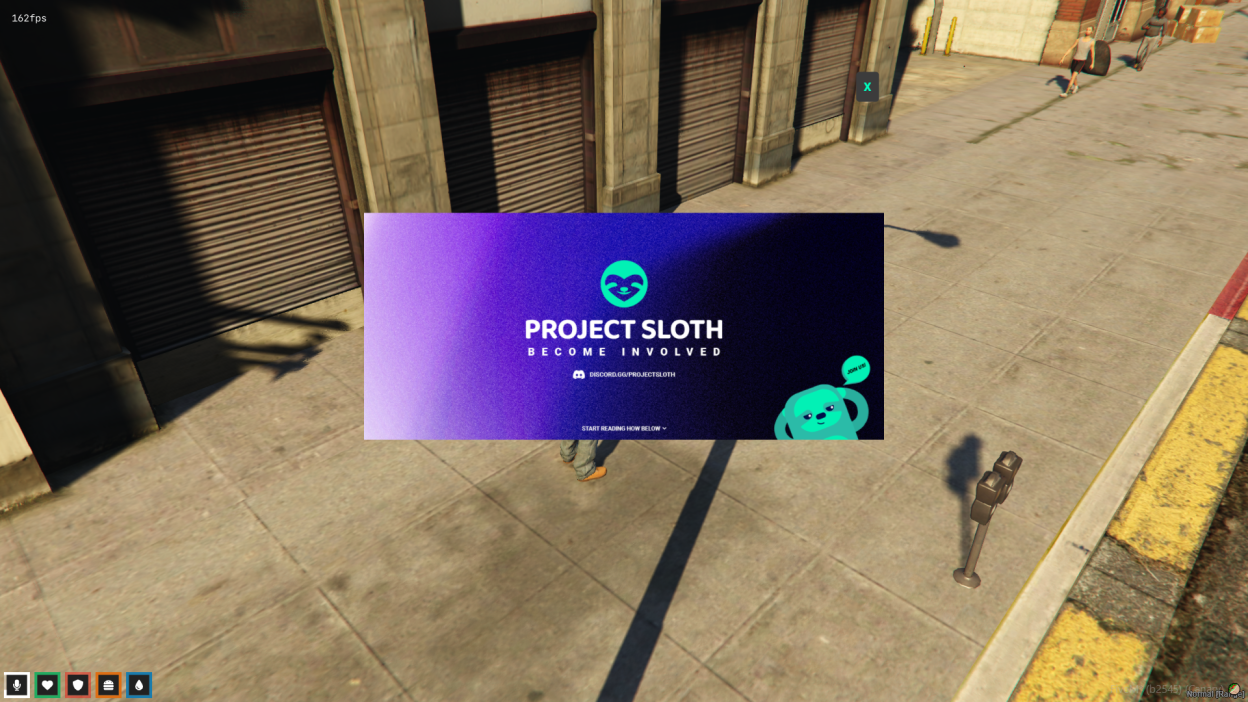
|
|
|
|
## Notify
|
|
|
|
```
|
|
exports['ps-ui']:Notify(text, texttype, length)
|
|
```
|
|
|
|
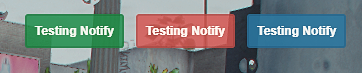
|
|
|
|
CREDITS:
|
|
- https://github.com/sharkiller/nopixel_minigame
|
|
- https://github.com/iLLeniumStudios/is-statushud
|
|
- https://github.com/tnj-development/tnj-notify
|